Bug
Through thorns to stars
Администратор
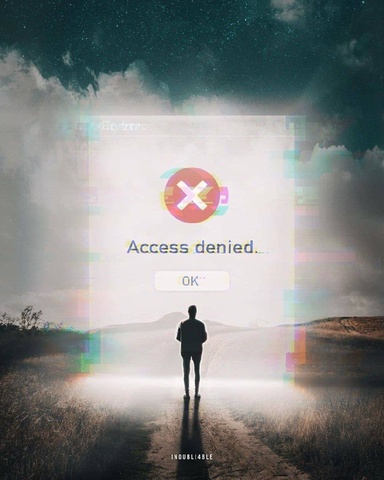
Bug
Through thorns to stars
Администратор
- Сообщения
- 1,491
- Реакции
- 2,945
C#:
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Threading;
using Leaf.xNet;
namespace Youtube_Livestream_Viewbot
{
internal class Program
{
private static void Main(string[] args)
{
Console.ForegroundColor = ConsoleColor.Green;
string value = "\r\n ▄█ █▄ ▄████████ ▄█ ▄███████▄ ███ █▄ \r\n███ ███ ███ ███ ███ ██▀ ▄██ ███ ███ \r\n███ ███ ███ █▀ ███ ▄███▀ ███ ███ \r\n███ ███ ▄███▄▄▄ ███ ▀█▀▄███▀▄▄ ███ ███ \r\n███ ███ ▀▀███▀▀▀ ███ ▄███▀ ▀ ███ ███ \r\n███ ███ ███ █▄ ███ ▄███▀ ███ ███ \r\n███ ▄█▄ ███ ███ ███ ███ ███▄ ▄█ ███ ███ \r\n ▀███▀███▀ ██████████ █▀ ▀████████▀ ████████▀ \r\n ";
Console.WriteLine(value);
Console.Write("Video ID = ");
string videoid = Console.ReadLine();
Console.Clear();
Console.WriteLine(value);
WebClient webClient = new WebClient();
webClient.DownloadFile("https://api.proxyscrape.com/?request=getproxies&proxytype=http&timeout=10000&country=all&ssl=all&anonymity=all", "proxyler.txt");
string text = "proxyler.txt";
bool flag = !text.Contains(".txt");
if (flag)
{
text += ".txt";
}
List<string> list = File.ReadLines(text).ToList<string>();
foreach (string proxyAddress in list)
{
ProxyClient prxy = HttpProxyClient.Parse(proxyAddress);
new Thread(delegate()
{
Program.BotLoop(videoid, prxy);
}).Start();
Thread.Sleep(30);
}
Thread.Sleep(-1);
Console.ReadLine();
}
private static void BotLoop(string videoid, ProxyClient proxy)
{
try
{
using (HttpRequest httpRequest = new HttpRequest())
{
httpRequest.UseCookies = true;
httpRequest.Proxy = proxy;
httpRequest.AddHeader("Host", "m.youtube.com");
httpRequest.AddHeader("Proxy-Connection", "keep-alive");
httpRequest.AddHeader("User-Agent", "Mozilla/5.0 (iPhone; CPU iPhone OS 13_3 like Mac OS X) AppleWebKit/605.1.15 (KHTML, like Gecko) Mobile/15E148 Instagram 123.1.0.26.115 (iPhone11,6; iOS 13_3; en_US; en-US; scale=3.00; 1242x2688; 190542906)");
httpRequest.AddHeader("Accept", "text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8");
httpRequest.AddHeader("Accept-Language", "ru-RU,ru;q=0.9,en-US;q=0.8,en;q=0.7");
httpRequest.AddHeader("Accept-Encoding", "gzip, deflate");
string text = httpRequest.Get("https://m.youtube.com/watch?v=" + videoid, null).ToString();
string text2 = text.Split(new string[]
{
"videostatsWatchtimeUrl\":{\"baseUrl\":\""
}, StringSplitOptions.None)[1].Split(new string[]
{
"\"}"
}, StringSplitOptions.None)[0].Replace("\\u0026", "&").Replace("%2C", ",").Replace("\\/", "/");
string text3 = text2.Split(new string[]
{
"cl="
}, StringSplitOptions.None)[1].Split(new char[]
{
'&'
})[0];
string text4 = text2.Split(new string[]
{
"ei="
}, StringSplitOptions.None)[1].Split(new char[]
{
'&'
})[0];
string text5 = text2.Split(new string[]
{
"of="
}, StringSplitOptions.None)[1].Split(new char[]
{
'&'
})[0];
string text6 = text2.Split(new string[]
{
"vm="
}, StringSplitOptions.None)[1].Split(new char[]
{
'&'
})[0];
httpRequest.AddHeader("Host", "s.youtube.com");
httpRequest.AddHeader("Proxy-Connection", "keep-alive");
httpRequest.AddHeader("User-Agent", "Mozilla/5.0 (iPhone; CPU iPhone OS 13_3 like Mac OS X) AppleWebKit/605.1.15 (KHTML, like Gecko) Mobile/15E148 Instagram 123.1.0.26.115 (iPhone11,6; iOS 13_3; en_US; en-US; scale=3.00; 1242x2688; 190542906)");
httpRequest.AddHeader("Accept", "image/png,image/svg+xml,image/*;q=0.8,video/*;q=0.8,*/*;q=0.5");
httpRequest.AddHeader("Accept-Language", "ru-RU,ru;q=0.8,en-US;q=0.5,en;q=0.3");
httpRequest.AddHeader("Referer", "https://m.youtube.com/watch?v=" + videoid);
Console.WriteLine("Botting with " + proxy.Host);
Program.BotsViewingStream++;
Console.Title = string.Format("Youtube View Bot by Weizu | Sending Bots | {0}", Program.BotsViewingStream);
int i = 0;
while (i <= 30)
{
try
{
for (;;)
{
httpRequest.Get(string.Concat(new string[]
{
"https://s.youtube.com/api/stats/watchtime?ns=yt&el=detailpage&cpn=isWmmj2C9Y2vULKF&docid=",
videoid,
"&ver=2&cmt=7334&ei=",
text4,
"&fmt=133&fs=0&rt=1003&of=",
text5,
"&euri&lact=4418&live=dvr&cl=",
text3,
"&state=playing&vm=",
text6,
"&volume=100&c=MWEB&cver=2.20200319.09.00&cplayer=UNIPLAYER&cbrand=apple&cbr=Safari%20Mobile&cbrver=12.1.15E148&cmodel=iphone&cos=iPhone&cosver=12_2&cplatform=MOBILE&delay=5&hl=ru&cr=GB&rtn=1303&afmt=140&lio=1556394045.182&idpj=&ldpj=&rti=1003&muted=0&st=7334&et=7634"
}), null).ToString();
i = 0;
Thread.Sleep(30000);
}
}
catch
{
i++;
Thread.Sleep(5000);
}
}
Console.WriteLine("Leaving with " + proxy.Host);
Program.BotsViewingStream--;
Console.Title = string.Format("Youtube View Bot by Weizu | Sending Bots | {0}", Program.BotsViewingStream);
}
}
catch
{
Console.Title = string.Format("Youtube View Bot by Weizu | Sending Bots | {0}", Program.BotsViewingStream);
}
}
private static int BotsViewingStream = 0;
}
}