Bug
Through thorns to stars
Администратор
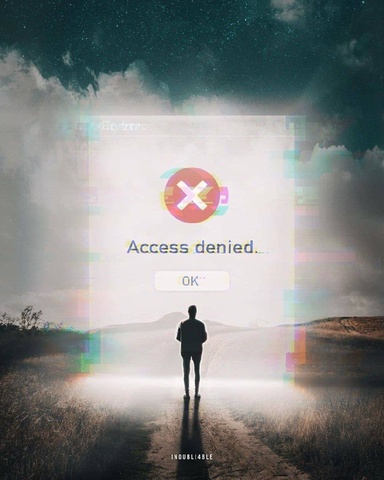
Bug
Through thorns to stars
Администратор
- Сообщения
- 1,491
- Реакции
- 2,939

VB.Net:
Код:
Public Class HeartBubbles
Inherits Control
Sub New()
SetStyle(ControlStyles.AllPaintingInWmPaint Or ControlStyles.UserPaint Or
ControlStyles.ResizeRedraw Or ControlStyles.OptimizedDoubleBuffer Or ControlStyles.SupportsTransparentBackColor, True)
Me.DoubleBuffered = True
Me.Size = New System.Drawing.Size(100, 400)
Me.BackColor = Color.Transparent
End Sub
Enum Bubble As Byte
Slow = 0
Fast = 1
End Enum
Public Shared Sync As Object = New Object()
Public Sub Add()
Add(Bubble.Slow, Nothing, Nothing)
End Sub
Public Sub Add(ByVal BubbleMode As Bubble, ByVal FillColor As Object, ByVal ColorBorder As Object)
Dim c0, c1 As Object
If FillColor Is Nothing Then
Dim r As New Random
c0 = Color.FromArgb(r.Next(255), r.Next(255), r.Next(255))
Else
c0 = FillColor
End If
If ColorBorder IsNot Nothing Then
c1 = ColorBorder
Else
c1 = Nothing
End If
Dim v0, v1, v2 As Integer
v0 = 17
v1 = 26
v2 = 3
SyncLock Sync
hearts.Add({c0, 245, CInt(Me.Width / 2) + rndm(3, 20), Me.Height - v1 + 10, v2, rndm(10, Me.Width - 10), -1, BubbleMode, v0, v1, c1})
End SyncLock
If Systimer0 Is Nothing Then
Systimer0 = New System.Timers.Timer
AddHandler Systimer0.Elapsed, AddressOf OnTimedEvent0
Systimer0.Interval = 1
Systimer0.AutoReset = True
End If
If Systimer0 IsNot Nothing Then
If Systimer0.Enabled = False Then
EnableTimer0()
End If
End If
End Sub
Private hearts As New List(Of Object)
Protected Overrides Sub OnPaint(e As PaintEventArgs)
Dim G As Graphics = e.Graphics
With G
.SmoothingMode = Drawing2D.SmoothingMode.AntiAlias
.PixelOffsetMode = Drawing2D.PixelOffsetMode.HighQuality
If hearts.Count > 0 Then
For Each i In hearts
Dim x As Object() = i
If x(4) >= x(8) And x(6) = 1 Then
x(4) = x(4) - 1
x(2) = x(2) + 1
ElseIf x(4) < x(9) And x(6) = -1 Then
x(4) = x(4) + 3
x(2) = x(2) - 1
Else
If x(4) >= x(9) And x(6) = -1 Then
x(6) = 1
Else
If x(6) = 1 Then
x(6) = 966
End If
End If
End If
Dim s As Integer = CInt(x(4))
Dim gp As Drawing2D.GraphicsPath = hGP(New Point(2, 2), New Point(s, s))
Using bm As New Bitmap(s + 12, s + 12)
Using gr As Graphics = Graphics.FromImage(bm)
gr.SmoothingMode = Drawing2D.SmoothingMode.AntiAlias
gr.PixelOffsetMode = Drawing2D.PixelOffsetMode.HighQuality
If x(1) > 0 Then
x(1) = x(1) - If(x(7) > 1, 3, 1)
End If
gr.FillPath(New SolidBrush(Color.FromArgb(CInt(x(1)), x(0).R, x(0).G, x(0).B)), gp)
If x(10) = Nothing Then
gr.DrawPath(New Pen(Color.FromArgb(CInt(x(1)), x(0).R, x(0).G, x(0).B), 1.6F), gp)
Else
gr.DrawPath(New Pen(Color.FromArgb(CInt(x(1)), x(10).R, x(10).G, x(10).B), 1.6F), gp)
End If
gr.Dispose()
End Using
Select Case DirectCast(x(7), Bubble)
Case Bubble.Slow
x(3) = x(3) - 1
Case Bubble.Fast
x(3) = x(3) - 2
End Select
.DrawImage(bm.Clone(), x(2), x(3))
bm.Dispose()
End Using
gp.CloseAllFigures()
Next
Dim rm As Object() = (From a As Object In hearts Select a Where a(1) <= 0).ToArray
If rm.Count > 0 Then
For Each i In rm
SyncLock Sync
hearts.Remove(i)
End SyncLock
Next
End If
End If
End With
End Sub
Private Function rndm(ByVal v0 As Integer, ByVal v1 As Integer) As Integer
Dim r As Random = New Random()
Return r.Next(v0, v1)
End Function
Private Function hGP(poi0 As Point, poi1 As Point) As Drawing2D.GraphicsPath
Dim w As Integer = Math.Max(poi1.X, poi0.X) - Math.Min(poi1.X, poi0.X)
Dim h As Integer = Math.Max(poi1.Y, poi0.Y) - Math.Min(poi1.Y, poi0.Y)
Dim a As Integer = CInt((w + h) / 2)
Dim hPath As New Drawing2D.GraphicsPath(Drawing2D.FillMode.Winding)
If a > 0 Then
Dim tlc As Point = poi0
If poi1.X < poi0.X Then
tlc.X = poi1.X
End If
If poi1.Y < poi0.Y Then
tlc.Y = poi1.Y
End If
Dim r As Integer = CInt(a / 2)
Dim y As Int32 = CInt(tlc.Y + r / 2 + Math.Sin(45 / 180 * Math.PI) * r / 2) + (r - (1 - Math.Sin(45 / 180 * Math.PI)) * r / 2)
hPath.AddArc(tlc.X, tlc.Y, r, r, 135.0F, 225.0F)
hPath.AddArc(tlc.X + r, tlc.Y, r, r, 180.0F, 225.0F)
hPath.AddLine(CInt(tlc.X + a - (1 - Math.Sin(45 / 180 * Math.PI)) * r / 2), CInt(tlc.Y + r / 2 + Math.Sin(45 / 180 * Math.PI) * r / 2), tlc.X + r, y)
hPath.CloseFigure()
End If
Return hPath
End Function
Private Systimer0 As System.Timers.Timer = Nothing
Private Sub DisableTimer0()
If Systimer0 IsNot Nothing Then
Systimer0.Stop()
Systimer0.Enabled = False
End If
End Sub
Private Sub EnableTimer0()
Systimer0.Enabled = True
End Sub
Private Sub OnTimedEvent0(source As Object, e As Timers.ElapsedEventArgs)
If hearts.Count = 0 Then
DisableTimer0()
Me.Invalidate()
Else
Me.Invalidate()
End If
End Sub
End Class
C#:
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Linq;
using System.Timers;
using System.Windows.Forms;
using Microsoft.VisualBasic.CompilerServices;
namespace Hearts
{
public class HeartBubbles : Control
{
public HeartBubbles()
{
base.SetStyle(ControlStyles.UserPaint | ControlStyles.ResizeRedraw | ControlStyles.SupportsTransparentBackColor | ControlStyles.AllPaintingInWmPaint | ControlStyles.OptimizedDoubleBuffer, true);
this.DoubleBuffered = true;
base.Size = new Size(100, 400);
this.BackColor = Color.Transparent;
}
public void Add()
{
this.Add(HeartBubbles.Bubble.Slow, null, null);
}
public void Add(HeartBubbles.Bubble BubbleMode, object FillColor, object ColorBorder)
{
bool flag = FillColor == null;
object c0;
if (flag)
{
Random r = new Random();
c0 = Color.FromArgb(r.Next(255), r.Next(255), r.Next(255));
}
else
{
c0 = FillColor;
}
bool flag2 = ColorBorder != null;
object c;
if (flag2)
{
c = ColorBorder;
}
else
{
c = null;
}
int v0 = 17;
int v = 26;
int v2 = 3;
object sync = HeartBubbles.Sync;
lock (sync)
{
this.hearts.Add(new object[]
{
c0,
245,
(int)((double)base.Width / 2.0) + this.rndm(3, 20),
base.Height - v + 10,
v2,
this.rndm(10, base.Width - 10),
-1,
BubbleMode,
v0,
v,
c
});
}
bool flag4 = this.Systimer0 == null;
if (flag4)
{
this.Systimer0 = new System.Timers.Timer();
this.Systimer0.Elapsed += this.OnTimedEvent0;
this.Systimer0.Interval = 1.0;
this.Systimer0.AutoReset = true;
}
bool flag5 = this.Systimer0 != null;
if (flag5)
{
bool flag6 = !this.Systimer0.Enabled;
if (flag6)
{
this.EnableTimer0();
}
}
}
protected override void OnPaint(PaintEventArgs e)
{
Graphics G = e.Graphics;
G.SmoothingMode = SmoothingMode.AntiAlias;
G.PixelOffsetMode = PixelOffsetMode.HighQuality;
bool flag = this.hearts.Count > 0;
if (flag)
{
foreach (object i in this.hearts)
{
object[] x = (object[])i;
bool flag2 = Conversions.ToBoolean(Operators.AndObject(Operators.ConditionalCompareObjectGreaterEqual(x[4], x[8], false), Operators.ConditionalCompareObjectEqual(x[6], 1, false)));
if (flag2)
{
x[4] = Operators.SubtractObject(x[4], 1);
x[2] = Operators.AddObject(x[2], 1);
}
else
{
bool flag3 = Conversions.ToBoolean(Operators.AndObject(Operators.ConditionalCompareObjectLess(x[4], x[9], false), Operators.ConditionalCompareObjectEqual(x[6], -1, false)));
if (flag3)
{
x[4] = Operators.AddObject(x[4], 3);
x[2] = Operators.SubtractObject(x[2], 1);
}
else
{
bool flag4 = Conversions.ToBoolean(Operators.AndObject(Operators.ConditionalCompareObjectGreaterEqual(x[4], x[9], false), Operators.ConditionalCompareObjectEqual(x[6], -1, false)));
if (flag4)
{
x[6] = 1;
}
else
{
bool flag5 = Conversions.ToBoolean(Operators.ConditionalCompareObjectEqual(x[6], 1, false));
if (flag5)
{
x[6] = 966;
}
}
}
}
int s = Conversions.ToInteger(x[4]);
GraphicsPath gp = this.hGP(new Point(2, 2), new Point(s, s));
using (Bitmap bm = new Bitmap(s + 12, s + 12))
{
using (Graphics gr = Graphics.FromImage(bm))
{
gr.SmoothingMode = SmoothingMode.AntiAlias;
gr.PixelOffsetMode = PixelOffsetMode.HighQuality;
bool flag6 = Conversions.ToBoolean(Operators.ConditionalCompareObjectGreater(x[1], 0, false));
if (flag6)
{
x[1] = Operators.SubtractObject(x[1], Conversions.ToBoolean(Operators.ConditionalCompareObjectGreater(x[7], 1, false)) ? 3 : 1);
}
gr.FillPath(new SolidBrush(Color.FromArgb(Conversions.ToInteger(x[1]), Conversions.ToInteger(NewLateBinding.LateGet(x[0], null, "R", new object[0], null, null, null)), Conversions.ToInteger(NewLateBinding.LateGet(x[0], null, "G", new object[0], null, null, null)), Conversions.ToInteger(NewLateBinding.LateGet(x[0], null, "B", new object[0], null, null, null)))), gp);
bool flag7 = Conversions.ToBoolean(Operators.ConditionalCompareObjectEqual(x[10], null, false));
if (flag7)
{
gr.DrawPath(new Pen(Color.FromArgb(Conversions.ToInteger(x[1]), Conversions.ToInteger(NewLateBinding.LateGet(x[0], null, "R", new object[0], null, null, null)), Conversions.ToInteger(NewLateBinding.LateGet(x[0], null, "G", new object[0], null, null, null)), Conversions.ToInteger(NewLateBinding.LateGet(x[0], null, "B", new object[0], null, null, null))), 1.6f), gp);
}
else
{
gr.DrawPath(new Pen(Color.FromArgb(Conversions.ToInteger(x[1]), Conversions.ToInteger(NewLateBinding.LateGet(x[10], null, "R", new object[0], null, null, null)), Conversions.ToInteger(NewLateBinding.LateGet(x[10], null, "G", new object[0], null, null, null)), Conversions.ToInteger(NewLateBinding.LateGet(x[10], null, "B", new object[0], null, null, null))), 1.6f), gp);
}
gr.Dispose();
}
HeartBubbles.Bubble bubble = (HeartBubbles.Bubble)x[7];
HeartBubbles.Bubble bubble2 = bubble;
if (bubble2 != HeartBubbles.Bubble.Slow)
{
if (bubble2 == HeartBubbles.Bubble.Fast)
{
x[3] = Operators.SubtractObject(x[3], 2);
}
}
else
{
x[3] = Operators.SubtractObject(x[3], 1);
}
bm.Dispose();
}
gp.CloseAllFigures();
}
object[] rm = (from a in this.hearts
select a).ToArray<object>();
bool flag8 = rm.Count<object>() > 0;
if (flag8)
{
foreach (object j in rm)
{
object sync = HeartBubbles.Sync;
lock (sync)
{
this.hearts.Remove(j);
}
}
}
}
}
private int rndm(int v0, int v1)
{
Random r = new Random();
return r.Next(v0, v1);
}
private GraphicsPath hGP(Point poi0, Point poi1)
{
int w = Math.Max(poi1.X, poi0.X) - Math.Min(poi1.X, poi0.X);
int h = Math.Max(poi1.Y, poi0.Y) - Math.Min(poi1.Y, poi0.Y);
int a = (int)((double)(w + h) / 2.0);
GraphicsPath hPath = new GraphicsPath(FillMode.Winding);
bool flag = a > 0;
if (flag)
{
Point tlc = poi0;
bool flag2 = poi1.X < poi0.X;
if (flag2)
{
tlc.X = poi1.X;
}
bool flag3 = poi1.Y < poi0.Y;
if (flag3)
{
tlc.Y = poi1.Y;
}
int r = (int)((double)a / 2.0);
int y = (int)((double)((int)((double)tlc.Y + (double)r / 2.0 + Math.Sin(0.78539816339744828) * (double)r / 2.0)) + ((double)r - (1.0 - Math.Sin(0.78539816339744828)) * (double)r / 2.0));
hPath.AddArc(tlc.X, tlc.Y, r, r, 135f, 225f);
hPath.AddArc(tlc.X + r, tlc.Y, r, r, 180f, 225f);
hPath.AddLine((int)((double)(tlc.X + a) - (1.0 - Math.Sin(0.78539816339744828)) * (double)r / 2.0), (int)((double)tlc.Y + (double)r / 2.0 + Math.Sin(0.78539816339744828) * (double)r / 2.0), tlc.X + r, y);
hPath.CloseFigure();
}
return hPath;
}
private void DisableTimer0()
{
bool flag = this.Systimer0 != null;
if (flag)
{
this.Systimer0.Stop();
this.Systimer0.Enabled = false;
}
}
private void EnableTimer0()
{
this.Systimer0.Enabled = true;
}
private void OnTimedEvent0(object source, ElapsedEventArgs e)
{
bool flag = this.hearts.Count == 0;
if (flag)
{
this.DisableTimer0();
base.Invalidate();
}
else
{
base.Invalidate();
}
}
public static object Sync = new object();
private List<object> hearts = new List<object>();
private System.Timers.Timer Systimer0 = null;
public enum Bubble : byte
{
Slow,
Fast
}
}
}
Для просмотра скрытого содержимого вы должны войти или зарегистрироваться.